Introduction
In ClickHouse, joins can significantly improve performance when working with large datasets. Joins allow you to combine data from multiple tables based on a common key, and perform various operations on the resulting combined data set.
Types of JOINS in ClickHouse
ClickHouse supports various types of joins, including:
- Inner join: returns only the rows with matching values in both tables.
- Left join: returns all the rows from the left table and matching rows from the right table. If there are no matching rows in the right table, the result will have NULL values for the columns from the right table.
- Right join: returns all the rows from the right table and matching rows from the left table. If there are no matching rows in the left table, the result will have NULL values for the columns from the left table.
- Full outer join: returns all the rows from both tables. If there are no matching rows in one of the tables, the result will have NULL values for the columns from that table.
Simple JOIN in ClickHouse
Here’s an example of how you can perform a join in ClickHouse:
-- create two sample tables CREATE TABLE table1 ( id Int32, name String, age Int32 ) ENGINE = MergeTree ORDER BY id; CREATE TABLE table2 ( id Int32, address String, phone String ) ENGINE = MergeTree ORDER BY id;
-- populate the tables with some data INSERT INTO table1 (id, name, age) VALUES (1, 'John', 25), (2, 'Mary', 30), (3, 'Bob', 40);INSERT INTO table2 (id, address, phone) VALUES (1, '123 Main St', '555-1234'), (3, '456 Elm St', '555-5678'), (4, '789 Oak St', '555-9012'); -- perform an inner join SELECT * FROM table1 INNER JOIN table2 ON table1.id = table2.id;
In this example, we’re creating two tables (table1 and table2) with different columns. We then populate the tables with some data using the INSERT statement.
Finally, we perform an inner join between the two tables based on their “id” columns, which are common between the two tables. The result of this join will include only the rows where there is a match between the “id” column in both tables.
Note that you can also perform joins using subqueries in ClickHouse, which can sometimes provide better performance. Here’s an example of a subquery join:
SELECT * FROM table1 WHERE id IN ( SELECT id FROM table2 WHERE address LIKE '%Main%' );
In this example, we’re using a subquery to find all the “id” values in table2 where the address contains the word “Main”. We then use these “id” values to filter the rows in table1, effectively performing a join between the two tables based on the “id” column.
Overall, joins are an important tool for working with large datasets in ClickHouse. By combining data from multiple tables, you can perform more complex operations and gain insights that might not be possible otherwise.
When working with joins in ClickHouse, there are several things to keep in mind to ensure good performance:
- Choose the right type of join: Different types of joins can have different performance characteristics, depending on the data and the query. In general, inner joins tend to be faster than outer joins, and subquery joins can sometimes be faster than regular joins.
- Use appropriate join keys: Join keys are the columns that are used to match rows between two tables. Choosing the right join keys is important for good performance. Ideally, join keys should be indexed, and they should have a low cardinality (i.e., a small number of distinct values).
- Consider denormalization: In some cases, it may be beneficial to denormalize your data by combining multiple tables into a single table. This can eliminate the need for joins altogether and improve query performance.
- Use appropriate data types: The data types of the join keys can also affect performance. For example, using a string data type for a join key can be slower than using an integer data type.
- Optimize your queries: Finally, it’s important to optimize your queries for performance. This can involve using appropriate indexes, minimizing the amount of data that needs to be read, and avoiding unnecessary computations.
Complex JOIN in ClickHouse (using subquery and left join)
Here’s an example of a more complex join in ClickHouse, using a subquery and a left join:
-- create two sample tables CREATE TABLE orders ( order_id Int32, customer_id Int32, order_date Date, total_price Float32 ) ENGINE = MergeTree ORDER BY order_id; CREATE TABLE customers ( customer_id Int32, first_name String, last_name String, email String ) ENGINE = MergeTree ORDER BY customer_id;
-- populate the tables with some data INSERT INTO orders (order_id, customer_id, order_date, total_price) VALUES (1, 1, '2022-01-01', 100), (2, 2, '2022-01-02', 200), (3, 1, '2022-01-03', 150); INSERT INTO customers (customer_id, first_name, last_name, email) VALUES (1, 'John', 'Doe', 'john@example.com'), (2, 'Jane', 'Smith', 'jane@example.com'), (3, 'Bob', 'Johnson', 'bob@example.com');
-- perform a left join with a subquery SELECT customers.first_name, customers.last_name, SUM(orders.total_price) AS total_spent FROM customers LEFT JOIN ( SELECT customer_id, total_price FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-01-31' ) AS orders ON customers.customer_id = orders.customer_id GROUP BY customers.first_name, customers.last_name;
In this example, we’re creating two tables (orders and customers) with different columns. We then populate the tables with some data using the INSERT statement.
Finally, we perform a left join between the customers table and a subquery that selects all the orders from January 2022. We use the “customer_id” column as the join key. The result of this join will include all the customers, even if they did not make any orders in January 2022. We then group the results by the customers’ first and last names, and calculate the total amount spent by each customer during January 2022.
Overall, joins are a powerful feature of ClickHouse that can help you work with large datasets more efficiently. By using appropriate join keys, choosing the right type of join, and optimizing your queries, you can take full advantage of ClickHouse’s performance capabilities.
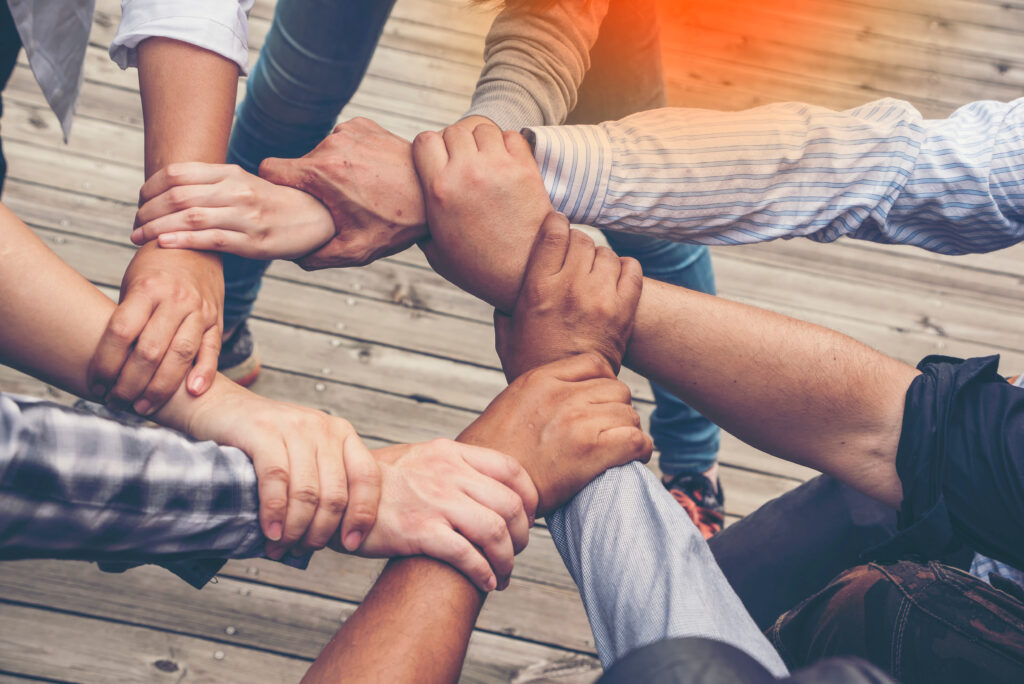
Considerations while using distributed tables with JOINs
One more thing to keep in mind when working with joins in ClickHouse is the use of distributed tables. ClickHouse supports distributed tables, which allow you to partition your data across multiple nodes in a cluster. This can improve performance by allowing queries to be executed in parallel across multiple nodes.
When using distributed tables with joins, there are a few additional considerations:
- Choose appropriate partition keys: When partitioning your data across nodes, you’ll need to choose an appropriate partition key. Ideally, this key should be used in the join condition to minimize data movement between nodes.
- Consider data skew: If your data is skewed (i.e., some partitions have significantly more data than others), this can affect join performance. You may need to use techniques like data shuffling or partitioning by a composite key to balance the data across nodes.
- Use appropriate replication: ClickHouse supports several replication strategies for distributed tables, including synchronous and asynchronous replication. Choosing the right replication strategy can affect both performance and data consistency.
Distributed JOIN in ClickHouse
Here’s an example of a distributed join in ClickHouse:
-- create a distributed table CREATE TABLE distributed_orders ( order_id Int32, customer_id Int32, order_date Date, total_price Float32 ) ENGINE = Distributed(cluster_name, default, orders, rand()); -- create a distributed table CREATE TABLE distributed_customers ( customer_id Int32, first_name String, last_name String, email String ) ENGINE = Distributed(cluster_name, default, customers, rand());
-- perform a distributed join SELECT customers.first_name, customers.last_name, SUM(orders.total_price) AS total_spent FROM distributed_customers AS customers LEFT JOIN distributed_orders AS orders ON customers.customer_id = orders.customer_id WHERE orders.order_date BETWEEN '2022-01-01' AND '2022-01-31' GROUP BY customers.first_name, customers.last_name;
In this example, we’re creating two distributed tables (distributed_orders and distributed_customers) that are partitioned across nodes in a cluster. We then perform a left join between the two tables using the “customer_id” column as the join key. We also filter the results to include only orders from January 2022.
By using distributed tables, we can take advantage of the parallel processing capabilities of ClickHouse to improve join performance. However, we’ll need to carefully choose our partition keys and replication strategies to ensure good performance and data consistency.
Another important consideration when working with joins in ClickHouse is the use of indexes. ClickHouse supports several types of indexes, including primary key indexes, secondary indexes, and bitmap indexes.
Indexes can significantly improve join performance by allowing ClickHouse to quickly locate the rows that need to be joined. However, indexes also come with some trade-offs, such as increased storage requirements and slower write performance.
Best practices for using indexes with JOINs in ClickHouse
Here are some best practices for using indexes with joins in ClickHouse:
- Use primary key indexes: If you have a primary key on your join key columns, ClickHouse will automatically use this index to perform the join. This can be much faster than a full table scan.
- Consider secondary indexes: If you don’t have a primary key on your join key columns, you can create a secondary index to improve join performance. However, keep in mind that secondary indexes can slow down write performance and increase storage requirements.
- Use bitmap indexes: Bitmap indexes can be useful for optimizing joins on columns with low cardinality (i.e., a small number of distinct values). Bitmap indexes can be much faster than regular indexes for these types of columns.
- Avoid unnecessary columns: When performing a join, it’s best to only select the columns that you actually need. This can reduce the amount of data that needs to be read and improve performance.
Secondary index for JOIN in ClickHouse
Here’s an example of using a secondary index for a join in ClickHouse:
-- create a table with a secondary index CREATE TABLE orders ( order_id Int32, customer_id Int32, order_date Date, total_price Float32 ) ENGINE = MergeTree ORDER BY order_id;
CREATE INDEX idx_customer_id ON orders (customer_id) TYPE minmax GRANULARITY 1;
-- perform a join using the secondary index SELECT * FROM orders INNER JOIN customers ON orders.customer_id = customers.customer_id;
In this example, we’re creating a table called “orders” with a secondary index on the “customer_id” column. We then perform an inner join between the “orders” table and a “customers” table based on the “customer_id” column. ClickHouse will automatically use the secondary index to perform the join, which can be much faster than a full table scan.
Conclusion
By using indexes effectively, we can improve join performance and take full advantage of ClickHouse’s speed and scalability. However, we’ll need to carefully balance the benefits of indexes against the potential trade-offs in terms of write performance and storage requirements.
To read more about ClickHouse JOINs, do consider reading the below articles